一个 简单的文本缩略+高亮 组件
# 图例
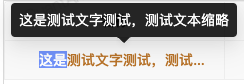
# props
- text
- 文本值
- highLightText
- 高亮文本值
- className
# 实现代码
点击 展开/收起 jsx 代码
/**
* @des 自动缩略文本组件
*/
import React, { useRef, useState } from 'react';
import * as _ from 'lodash';
import { Tooltip } from 'antd';
import Highlight from './HighLight';
const Abbr = ({ text, className, highLightText }) => {
const spanRef = useRef(null);
const [visible, setVisible] = useState(false);
const handleCheckOver = () => {
if (spanRef.current) {
return spanRef.current.scrollHeight > spanRef.current.clientHeight;
}
return false;
};
const handleVisibleChange = (vis) => {
if (vis) {
if (handleCheckOver()) {
setVisible(true);
}
} else {
setVisible(false);
}
};
return (
<Tooltip open={visible} onOpenChange={handleVisibleChange} title={text}>
<span
ref={spanRef}
style={{
WebkitLineClamp: 1,
lineHeight: 'inherit',
display: '-webkit-box',
overflow: 'hidden',
whiteSpace: 'pre-wrap',
wordBreak: 'break-all',
'-webkit-box-orient': 'vertical',
hyphens: 'auto',
}}
className={className ?? ''}
>
<Highlight text={text} highLightText={highLightText} />
</span>
</Tooltip>
);
};
export default Abbr;
/**
* @des 高亮文本组件
*/
import React from 'react';
import * as _ from 'lodash';
const Highlight = ({ text, highLightText }) => {
if (!highLightText) {
return <span>{text}</span>;
}
if (!_.isString(text)) {
return <span>{text}</span>;
}
const parts = text.split(new RegExp(`(${highLightText})`, 'gi'));
return (
<span>
{parts.map((part, index) =>
part.toLowerCase() === highLightText.toLowerCase() ? (
<span
key={index}
style={{ backgroundColor: 'var(--brand80)', color: 'var(--white)' }}
>
{part}
</span>
) : (
<span key={index}>{part}</span>
)
)}
</span>
);
};
export default Highlight;